Yo ✌
A lot of product managers can be code illiterate but you don’t have to be a developer to make use of some handy features in Slack. As long as you’ve used a computer before you should be able to follow along and impress your team with a bot that does something useful (or not).
I’m not a fan of Slack anymore and prefer Discord but a lot of organisations are using it. Anyway, the process to make a bot for Discord or Microsoft Teams is similar.
Since sometime in 2018 your Slack admins can download private conversations via “compliance exports”. Private messages and channels aren’t actually private. Not that anyone should be using the internets to communicate anything they want to keep a secret.
What you’ll need to make a bot
- Slack account with privileges
- Some kind of code editor, like Visual Studio Code or Jupyter for using Python
- Courage, I’ll explain all the steps and keep the code simple so don’t worry if you aren’t a developer
Writing a bot
Set up a Python environment if you don’t have one : https://freshprinceofstandarderror.com/ai/setting-up-your-data-science-environment/
We’ll make a bot that looks at a public Google sheet and notifies some Slack channel each time it is updated.
Make a Google Sheet and click share to make it viewable by anyone who has the link.
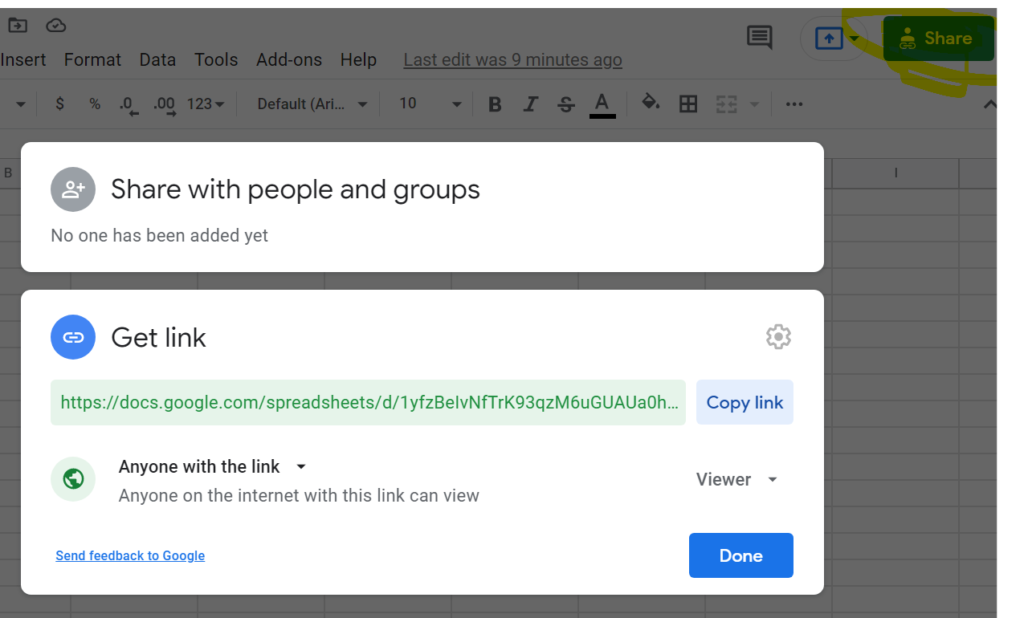
A trick to reading any Google sheet in Pandas is to append ‘/export?format=csv&gid=’ after the / following your unique URL ID. This will avoid a bunch of other hassles such as using the Google API.
import pandas as pd
#Replace g_sheet with your own Google Sheet URL
g_sheet = 'https://docs.google.com/spreadsheets/d/1yfzBeIvNfTrK93qzM6uGUAUa0hb3zu08cXGsDH-LKNc/edit#gid=0'
g_csv = g_sheet.replace('/edit#gid=', '/export?format=csv&gid=')
df = pd.read_csv(g_csv)
display(df)
Sweet, now the DataFrame should update with new values each time you run that cell.
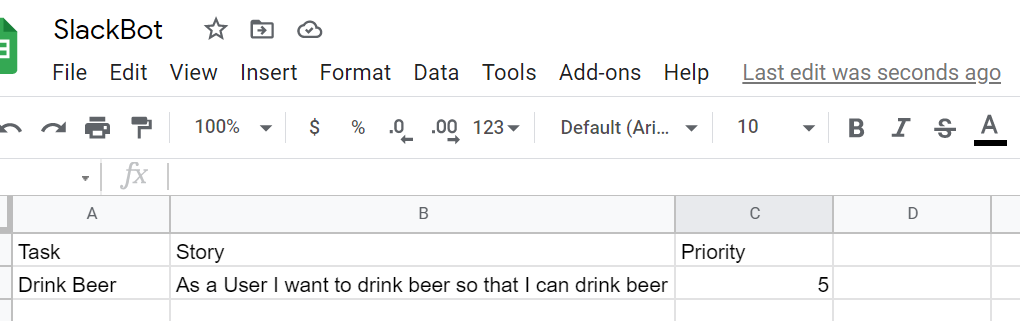
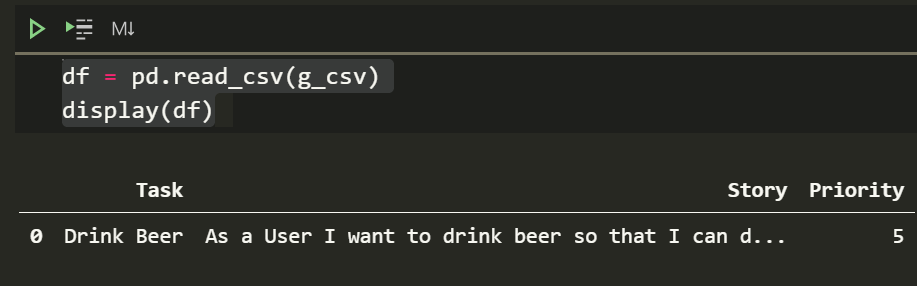
We can make a simple function that returns the last row of the DataFrame as a string later.
Get a Slack Webhook URL
Go to this URL: https://api.slack.com/messaging/webhooks
Click the create your Slack app button there. It should take you to this page.
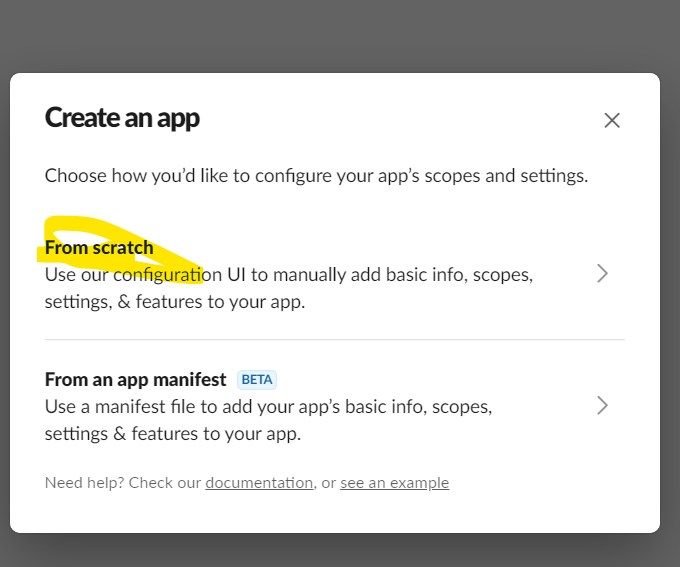
Select the App name and workspace
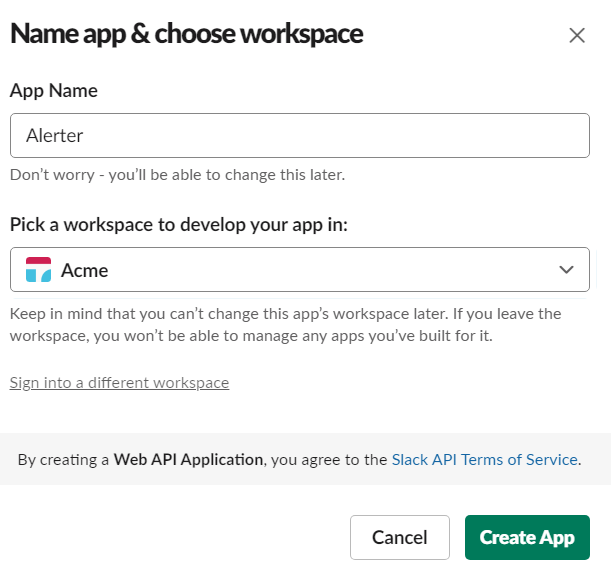
The next page should have a bunch of features and functionality. We want to add an incoming Webhook.
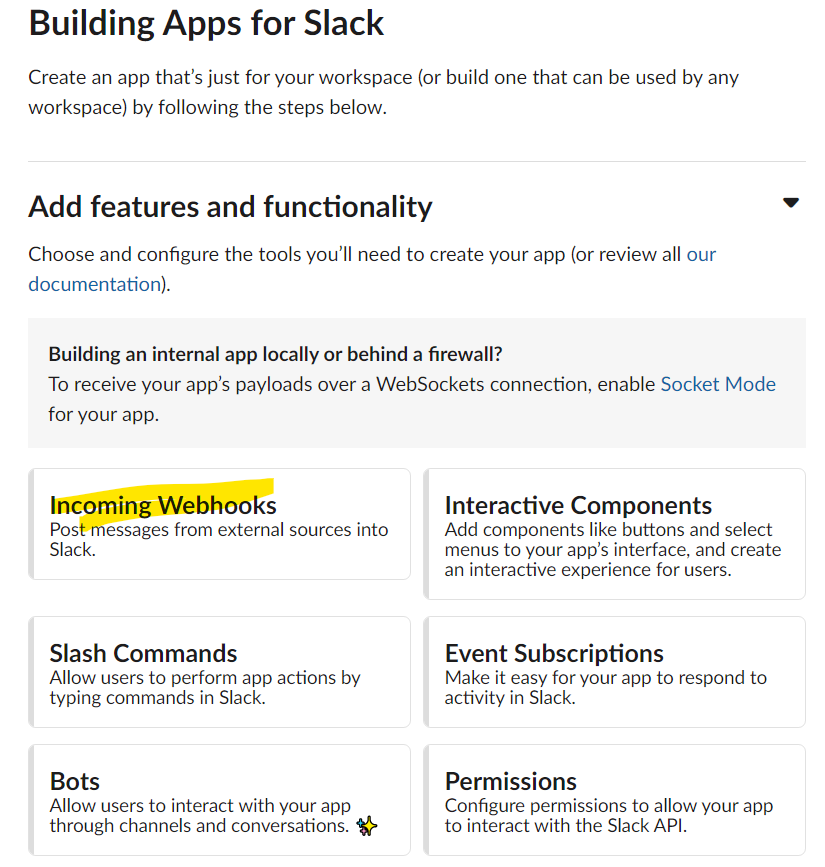
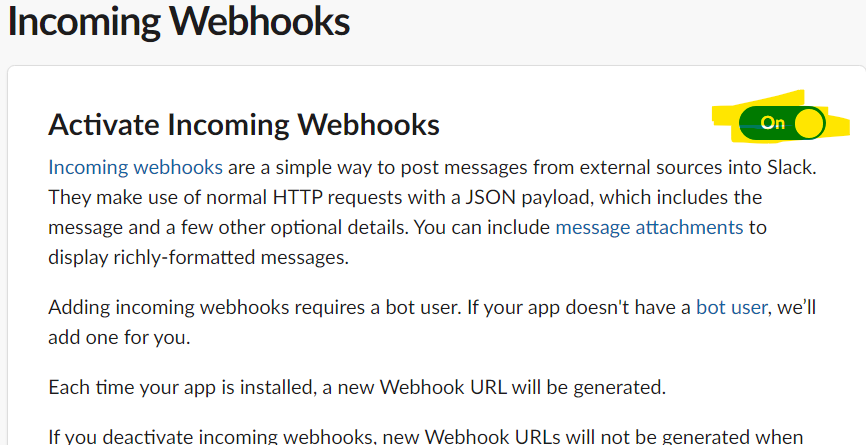
After switching on Webhooks a new option should appear at the bottom. Click to add a new Webhook and pick the appropriate channel.
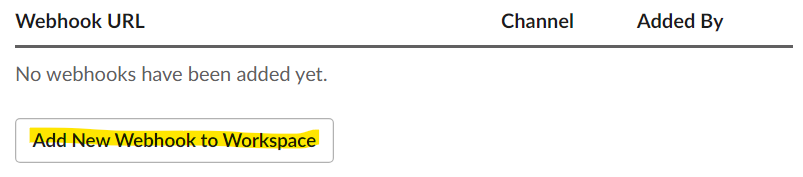
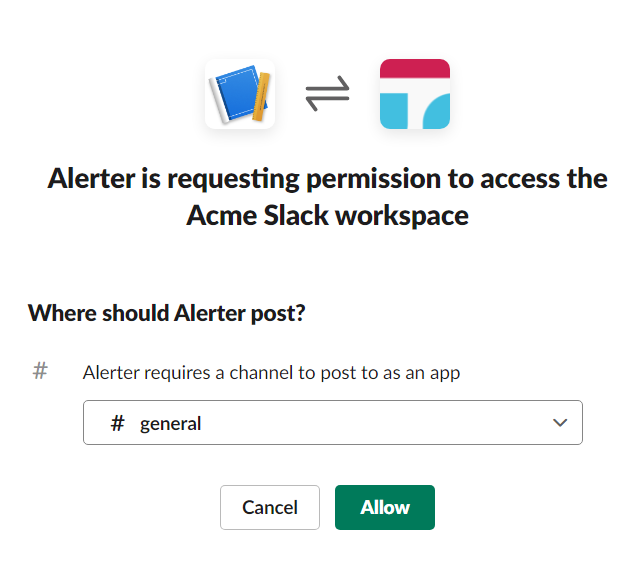
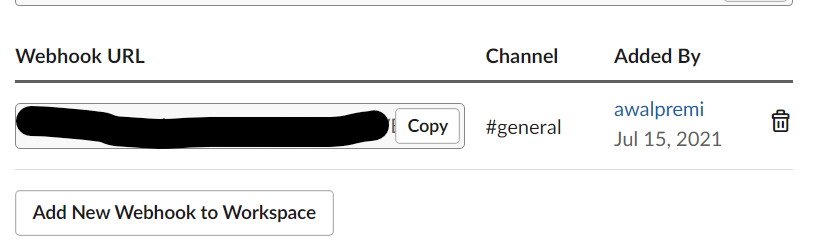
Now it should take you back and give you the URL which is a huge secret. So try not to share it unless you want “hackers” ? to post into your Slack. If Slack finds the URL somewhere public they block its use.
Back to writing the Bot
Copy and paste the URL into another cell
#Replace it with your own URL
WEBHOOK_URL= 'https://hooks.slack.com/BLABLA'
Import requests and test if everything is working.
import requests
pd.set_option("display.max_colwidth", None)
message = df.iloc[-1].to_string()
payload = {'text':message}
requests.post(WEBHOOK_URL, json=payload)
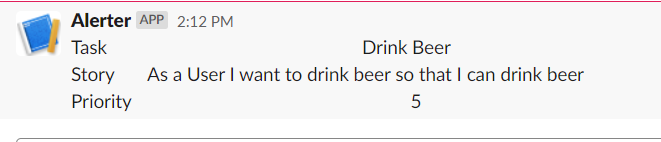
We can clean it all up and put it into a small .PY file.
import pandas as pd
import requests
pd.set_option("display.max_colwidth", None)
g_sheet = 'https://docs.google.com/spreadsheets/d/1yfzBeIvNfTrK93qzM6uGUAUa0hb3zu08cXGsDH-LKNc/edit#gid=0'
g_csv = g_sheet.replace('/edit#gid=', '/export?format=csv&gid=')
WEBHOOK_URL = 'INSERT YOUR URL'
def create_message():
df = pd.read_csv(g_csv)
message = df.iloc[-1].to_string()
return message
def push_message(message):
payload = {'text':message}
requests.post(WEBHOOK_URL, json=payload)
push_message(create_message())
Add another row to the spreadsheet and test it again.
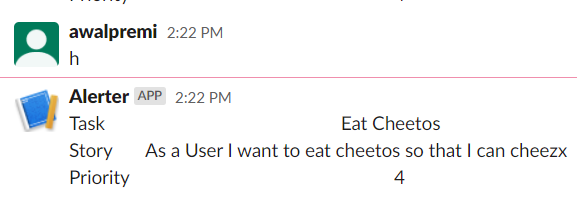
Nice!
What you learned
- Writing a neat script
- Interfacing with Slack via Webhook
- Reading data from Google Sheets easily
- It’s not perfect but now that you understand the process to go forth and experiment
Next steps would be having it run in the cloud in some sort of loop or cron job which I’ll write about in the following article: